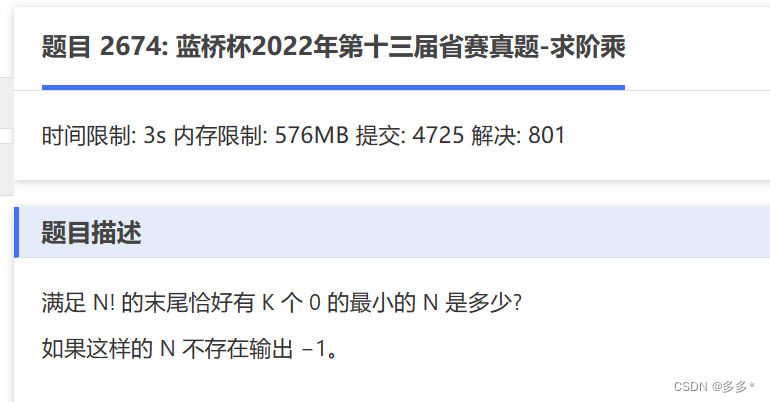
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
long n= sc.nextLong();
//设置双指针
long i=1;
long j=Long.MAX_VALUE;
//标准二分查找法 时间复杂度为log2n
while(i<j){
//位运算符防止溢出
long m=(i+j)>>>1;
if(n<=judge(m))
j=m;//右指针左移
else i=m+1;//左指针右移
}
if(judge(j)==n) System.out.println(j);
else System.out.println("-1");
}
public static long judge(long n){
//寻找n的阶乘中0后缀的数量
long cnt=0;
/*
* 寻找阶乘后有多少个0 2*5为0
* 看拆分为多个个5
* 就是多少个0
* 25可以拆成5*5
* 所以要对n一直除5
* */
while(n>0){
cnt+=n/5;
n/=5;
}
return cnt;
}
}